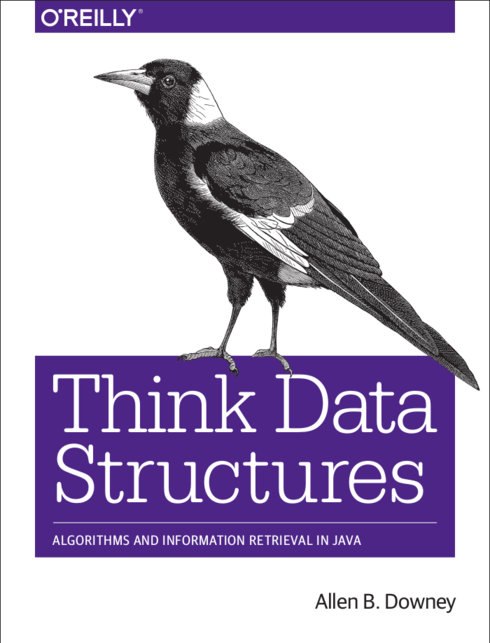
Think Data Structures: Algorithms and Information Retrieval in Java
Allen B. Downey
Copyright Year:
Publisher: Green Tea Press
Language: English
Formats Available
Conditions of Use
Attribution-NonCommercial-ShareAlike
CC BY-NC-SA
Reviews





This book is a great introduction to data structures and algorithms in Java, and covers all of the points I would want to introduce to a first-year Computer Science student. read more
This book is a great introduction to data structures and algorithms in Java, and covers all of the points I would want to introduce to a first-year Computer Science student.
This book has a good grasp on the material being introduced, and provides an accurate and unbiased perspective on how to implement and profile data structures in Java.
The Java-based examples in the book using modern programming techniques, and the GitHub repository associated with the book would be easy to update if new features were released that would enhance the material being introduced.
The organization of the material is intuitive, and introduces the material at the appropriate level for the target audience.
The book uses Java examples throughout, and is consistent in its approach.
The organization of the book is intuitive, and allows for a build up of understanding as you progress through the material. Sections are appropriately sized, and should be easy to follow for students.
The material is presented in a logical fashion that would make it easy to follow along with in a classroom.
The content is readable and does not seem to have any issues in the presentation of graphics or bookmarks.
The book does not have any obvious grammatical errors.
Although I have not yet worked through every example in the book, the ones that I have interacted with are presented in a very neutral way that are not insensitive to the audience.
I am going to be adopting this book for my summer data structures course in Java, and I am excited to be able to find such a high-quality OER resource on the site!





This book is intended for a Data Structures in Java course that has a prerequisite of students having basic Java knowledge. With this viewpoint, it does a good job in coming straight to the point by starting where most CS2 or follow-up advanced... read more
This book is intended for a Data Structures in Java course that has a prerequisite of students having basic Java knowledge. With this viewpoint, it does a good job in coming straight to the point by starting where most CS2 or follow-up advanced Java courses begin. The author has done a good job in delving in optimal mathematical component that is required while doing algorithm analysis. Students usually learn associated mathematical concepts in other courses like "Discrete Structures". Author does not devote separate sections or chapters for introducing Stacks and Queues as is usually seen in similar Data Structures books, but briefly introduces them during tree traversal, where they are actually applied in line with author's "top-down" approach. I liked this approach, but a little more elaboration on all the structures and their relative applications through examples would help.
I did not find any errors in the text or the code on GitHub.
It is not possible for a technical book to stay relevant for a long time unless new editions come in frequently. New Java versions come out at regular intervals. But, data structures concepts and their corresponding exercises in Java remain the same. A major new addition that has been introduced in new versions of Java is the lambda expression, and instructors can give these as added exercises to the students to explore and implement on their own. The author has used JUnit tests which also have been written in a previous version of JUnit4. A new edition of this book could also incorporate code changes as per JUnit5 - Jupiter tests.
The author's writing style is clear and easy to read. Also, hyperlinks have been included at appropriate places as references for students to fall back on and revise/clarify any concept.
The text is consistent and follows a "Top-down approach" as has been advocated by the author himself. Furthermore, the text is consistent from chapter to chapter and within chapters. I liked the way the author starts off with the example of Selection Sort and comes a full circle by finishing the text with a demonstration and an analysis of remaining sorting algorithms.
The text is highly modular, and the reader should be able to proceed from chapter to chapter. Writing JUnit tests advocates good “Test Driven Development” practice in students. Also, author has given access to code on GitHub which is another plus point.
Organization of the text follows a "Top-down approach". The author has taken a different approach and has done a good job in explaining the material. Given the audience is well-versed with Java fundamentals, they would enjoy reading the text as the exercises seamlessly flow from chapter to chapter.
The instructor may have to design additional exercises or refer to other course materials to explain topics that would require an in-depth "treatment" in a Data Structures class like Stacks, Queues, Circular Queues, B-trees, Graphs, etc. Hence, this textbook can be used as a Supplemental resource, rather than a full-fledged recommended textbook for a course.
It is available as both, in pdf format as well as in HTML, in the free version. Both are well-formatted. The hyperlinks are available in both formats at appropriate places to fall back on and revise/clarify any concept, which makes it very convenient. Moreover, availability of entire code on GitHub is an added advantage.
There are no grammatical errors. I did not find any!
The text was not insensitive or offensive. It did not use any examples that were non-inclusive.
Overall, I liked the flow of the text and the different approach taken by the author. The author has used the mathematical component to the optimal level as he advocated. Analysis of algorithms based on their implementations via ArrayList versus LinkedList and the usage of profiler to demonstrate their real-time output is a good way of comparison. The Wikipedia example and the inclusion of Redis are good sources for both undergraduate and graduate students.





While this book covers most of the major topics (linked lists, stacks, queues, binary trees, graphs, searching, sorting, asymptotic complexity analysis) of an introductory data structures book, it does so in an unconventional way. The author made... read more
While this book covers most of the major topics (linked lists, stacks, queues, binary trees, graphs, searching, sorting, asymptotic complexity analysis) of an introductory data structures book, it does so in an unconventional way. The author made a conscious choice to keep the textbook shorter and focus on the major aspects that software developers should learn. As a result, on the positive side, the book is short enough that it's possible that students might actually read the whole thing. Furthermore, this textbook includes additional concepts and material (e.g. about how web search engines work, and data persistence using a database). The book is very Java-centric, using real Java code examples throughout, rather than pseudocode. I like that approach, but it would only work well for students who had previous experience with Java. I did find, however, that some topics were introduced (like depth-first search), but barely elaborated on. Similarly, there were subtopics missing. The textbook's hashmap implementation was essentially using the “separate chaining” method for collision resolution (although it wasn't described by that name), and there was no mention of alternative approaches such as linear/quadratic probing. double-hashing, etc. Priority queues & heaps were mentioned and the ideas of how they work was sketched out, but implementation details weren't really discussed.
I did not find any mistakes/errors. In the textbook, the author expresses a slight bias against traditional data structures/algorithms courses for spending too much time analyzing sorting algorithms, and perhaps for taking an overly theoretical approach in general. Thus, on the “applied” to “theoretical” spectrum, this textbook falls very strongly in the applied camp. There were times when the book felt like it was equal parts “software development” and “data structures”. This might work well for places looking to reinforce (or introduce) software development skills throughout more courses within their curriculum. On the other hand, this textbook probably wouldn't serve as well for CS majors who will pursue graduate school in theoretical computer science, as they would need to be exposed to a more mathematically rigorous treatment of data structures. That material, however, could be offered in an upper level Algorithms course with a discrete mathematics prerequisite.
Staying entirely up-to-date is always a challenge for computer science textbooks, especially if they choose to use an industry language (like Java), rather than pseudocode. New version of Java are now coming out every 6 months. That said, I think this textbook will have a reasonable longevity. This text was written using JDK 7, so it doesn't include some more recent Java features (like lambda expressions or the streams API), but it does make good use of Java's generic types when implementing data structures. Once or twice, I felt that the careful use of correct generic type declarations (e.g. “Comparable”) impeded accessibility of the concepts being explained. However, I can see the argument that it is useful for students to learn how the generic/parameterized type system works in Java.
In general, I found the author's writing clear and easy to follow. The author conveys ideas with a minimum of jargon, introducing technical terms as they appear.
That said, there were some places where I felt the exposition could be improved. For instance, the first code example (ListClientExample) felt too abstract. In my experience, students learn better when they can see concrete examples first, rather than generalizations, and thus a simple (but specific) example such as a a “grocery list” (where the elements were each Strings) would have worked better. I also took exception with the author's casual use of a FOR loop to find the end of a linked list [ for ( ; node.next != null; node = node.next) {} ], when a WHILE loop seems much more appropriate. However, this is arguably a matter of taste/style. The author also reinforced many good software development habits, such as extensive use of unit tests, which is a welcome addition to a data structures textbook targeted at computer science majors who would like to become professional programmers.
This textbook used consistent terminology throughout, defining terms that might be new to students. Furthermore, I laud the author on consistently including short clickable hyperlinks to additional web resources where the student could learn more about a given topic if they wished.
The textbook is divided into reasonably-sized chapters. There is a considerable “flow” to the book, and I would recommend that it should generally be read in-order, rather than attempting to skip around to different chapters. This is especially true since the programming exercises at the end of each chapter are often answered within the text of the following chapter. (Instructors will need to come up with their own exercises/assignments to accompany this text – but that is almost universally true, since even when textbook provide a large collection of exercises, answer keys inevitably end up out on the web.)
Yes, there is a clear and logical order to the book. It is somewhat unconventional, because the sometimes the data structures, algorithms, or analysis techniques are introduced in the context where they are needed (e.g. motivated by the web crawler or search engine indexing application), rather than organized more clinically. Bits of theory are interwoven with the applications in a way that I think makes sense. However, this textbook would serve poorly as a “reference book” – rather, it is designed to be an educational journey/experience.
I was able to read it well as a PDF on both my laptop and my phone (not ideal that small, but it was very workable). The textbook is well formatted, and the occasional figures that were included were very helpful in supporting the text.
I did not notice any grammatical errors. Good quality writing/editing!
I did not notice anything culturally insensitive in this book. I thought the discussion of the “Getting to Philosophy” conjecture about Wikipedia was an interesting/relevant inclusion of some popular/Internet culture, which I think would be interesting to students from many backgrounds. The author did include one remark about how the code would need to be modified to work with Wikipedia pages using languages other than English, which I though showed at least a modicum of consideration for diverse readership.
Overall, I am impressed by the amount of effort the Allen Downey has put into not only this book, but the whole “Think X” series of books. It is remarkable how prolific he is, and how he provides a continual stream of high quality educational resources to the community, entirely for free!





This book is intended for a CS 2 course. Despite its being shorter than other CS 2 books I am familiar with, this book covers key topics commonly found in CS 2 courses. See the "Organization" section for comments that also relate to... read more
This book is intended for a CS 2 course. Despite its being shorter than other CS 2 books I am familiar with, this book covers key topics commonly found in CS 2 courses. See the "Organization" section for comments that also relate to comprehensiveness.
I did not detect any errors in the text. However, I examined only a few code references to code on GitHub or the author's website so I cannot comment on that code.
For example, Chapter 15, Crawling Wikipedia, describe interfaces to Wikipedia in detail. If that interface were to change through enhancement, portions of the chapter might have to be revised.
The author's writing style is easy to read, in fact a pleasure to read.
References to different parts of the text are consistent and reliable. I found only one variable that was not named according to accepted Java naming conventions (elt_i in Section 17.1).
The text is highly modular and easily broken down into sub-units that can be covered in a class session. This made the text easy to read in absorbable parts.
*Excellent* organization in which topics follow easily from one another. A big strength of the book is its use of GitHub and the author's website to store code that would otherwise be included in the text, reducing the modularity. Other CS 2 textbooks are bloated with code that could be moved elsewhere without interfering with the flow. This textbook achieves that.
The description of "Interface" matches the contents of the book.
I did not find one grammatical error.
There are no examples in the book that could be considered non-inclusive.
Overall:
* Exercises are well-spaced.
* There is a shortage of short, quick exercises for immediate practice and reinforcement.
* There is a comprehensive index.
* The book begins with some advanced-for-CS 2 concepts but the author explains most of them.
* Chapter 14 introduces Redis, giving CS 2 students an early introduction to database concepts.
* Chapter 17 on sorting rightly begins with, "Computer science departments have an unhealthy obsession with sort algorithms."
Table of Contents
- Chapter 1: Interfaces
- Chapter 2: Analysis of Algorithms
- Chapter 3: ArrayList
- Chapter 4: LinkedList
- Chapter 5: Doubly-linked list
- Chapter 6: Tree traversal
- Chapter 7: Getting to Philosophy
- Chapter 8: Indexer
- Chapter 9: The Map interface
- Chapter 10: Hashing
- Chapter 11: HashMap
- Chapter 12: TreeMap
- Chapter 13: Binary search tree
- Chapter 14: Persistence
- Chapter 15: Crawling Wikipedia
- Chapter 16: Boolean search
- Chapter 17: Sorting
Ancillary Material
About the Book
Data structures and algorithms are among the most important inventions of the last 50 years, and they are fundamental tools software engineers need to know. But in my opinion, most of the books on these topics are too theoretical, too big, and too bottom-up:
- Too theoretical: Mathematical analysis of algorithms is based on simplifying assumptions that limit its usefulness in practice. Many presentations of this topic gloss over the simplifications and focus on the math. In this book I present the most practical subset of this material and eliminate the rest.
- Too big: Most books on these topics are at least 500 pages, and some are more than 1000. By focusing on the topics I think are most useful for software engineers, I kept this book under 250 pages.
- Too bottom-up: Many data structures books focus on how data structures work (the implementations), with less about how to use them (the interfaces). In this book, I go “top down”, starting with the interfaces. Readers learn to use the structures in the Java Collections Framework before getting into the details of how they work.
Finally, many present this material out of context and without motivation: it’s just one damn data structure after another!
I try to alleviate the boredom by organizing the topics around an application—web search—that uses data structures extensively, and is an interesting and important topic in its own right.
This application also motivates some topics that are not usually covered in an introductory data structures class, including persistent data structures, with Redis, and streaming algorithms.
This book also presents basic aspects of software engineering practice, including version control and unit testing. Each chapter ends with an exercise that allows readers to apply what they have learned. Each exercise includes automated tests that check the solution. And for most exercises, I present my solution at the beginning of the next chapter.
This book is intended for college students in computer science and related fields, as well as professional software engineers, people training in software engineering, and people preparing for technical interviews.
I assume that the reader knows Java at an intermediate level, but I explain some Java features along the way, and provide pointers to supplementary material.
People who have read Think Java or Head First Java are prepared for this book.
About the Contributors
Author
Allen B. Downey is an American computer scientist, professor of computer science at the Franklin W. Olin College of Engineering and writer of free textbooks.
Downey received in 1989 his BS and in 1990 his MA, both in Civil Engineering from the Massachusetts Institute of Technology, and his PhD in Computer Science from the University of California at Berkeley in 1997.
He started his career as Research Fellow in the San Diego Supercomputer Center in 1995. In 1997 he became Assistant Professor of Computer Science at Colby College, and in 2000 at Wellesley College. He was Research Fellow at Boston University in 2002 and Professor of Computer Science at the Franklin W. Olin College of Engineering since 2003. In 2009-2010 he was also Visiting Scientist at Google Inc.